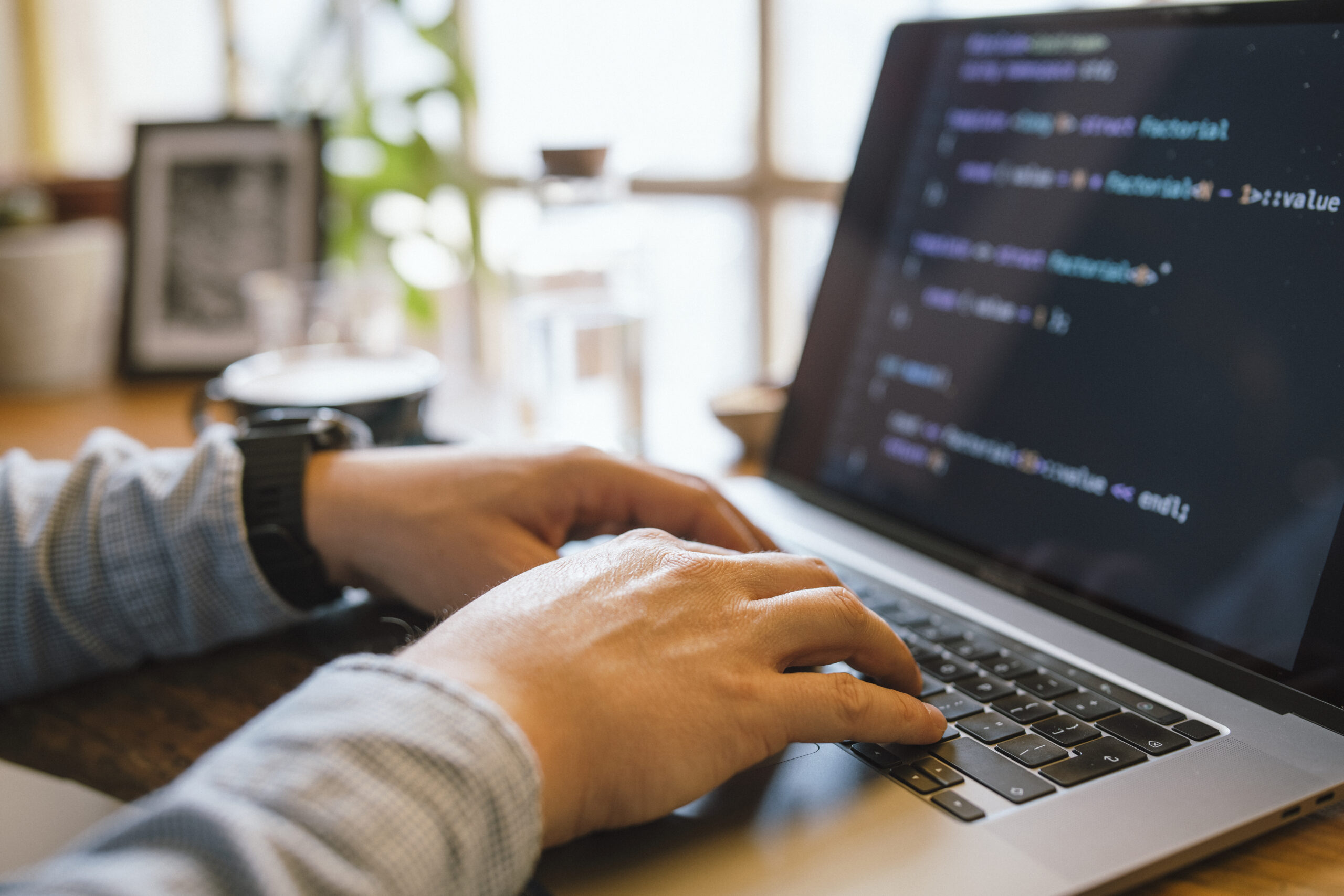
Debugging is One of the more important — nevertheless normally overlooked — expertise in the developer’s toolkit. It isn't really pretty much correcting damaged code; it’s about comprehending how and why items go Improper, and Finding out to Assume methodically to unravel challenges competently. Whether you are a starter or simply a seasoned developer, sharpening your debugging skills can help you save several hours of irritation and radically improve your efficiency. Here are several strategies to help builders stage up their debugging match by me, Gustavo Woltmann.
Grasp Your Resources
Among the quickest means builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although creating code is 1 part of enhancement, recognizing tips on how to communicate with it successfully all through execution is Similarly crucial. Contemporary enhancement environments appear equipped with powerful debugging abilities — but a lot of developers only scratch the area of what these equipment can do.
Choose, by way of example, an Integrated Improvement Environment (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These tools assist you to set breakpoints, inspect the worth of variables at runtime, phase via code line by line, and perhaps modify code to the fly. When employed correctly, they Enable you to observe just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-stop builders. They allow you to inspect the DOM, keep an eye on community requests, check out serious-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert frustrating UI troubles into workable tasks.
For backend or program-stage builders, resources like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command more than managing procedures and memory administration. Studying these equipment can have a steeper learning curve but pays off when debugging general performance problems, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be aware of code history, discover the exact second bugs have been launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default options and shortcuts — it’s about building an intimate understanding of your growth natural environment making sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you are aware of your applications, the greater time you could expend resolving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most crucial — and often overlooked — ways in helpful debugging is reproducing the situation. In advance of jumping in to the code or making guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. With out reproducibility, correcting a bug will become a recreation of opportunity, often bringing about wasted time and fragile code modifications.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire queries like: What steps resulted in the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you've, the a lot easier it turns into to isolate the precise problems under which the bug takes place.
After you’ve gathered ample information, endeavor to recreate the trouble in your neighborhood atmosphere. This may imply inputting a similar info, simulating identical consumer interactions, or mimicking process states. If the issue appears intermittently, consider composing automatic exams that replicate the sting conditions or condition transitions included. These tests not merely enable expose the issue and also prevent regressions Later on.
From time to time, the issue could possibly be environment-certain — it'd happen only on specific running systems, browsers, or below distinct configurations. Working with tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t merely a action — it’s a mentality. It requires persistence, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. With a reproducible circumstance, You should utilize your debugging applications more effectively, test possible fixes safely, and communicate much more clearly with your team or users. It turns an summary criticism right into a concrete problem — and that’s in which developers thrive.
Study and Comprehend the Error Messages
Mistake messages in many cases are the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really master to take care of mistake messages as direct communications from the procedure. They normally inform you just what occurred, where it transpired, and often even why it occurred — if you know the way to interpret them.
Get started by looking at the concept carefully As well as in total. Many builders, particularly when under time force, glance at the main line and quickly begin earning assumptions. But deeper in the mistake stack or logs might lie the legitimate root lead to. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them 1st.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or possibly a logic error? Will it point to a particular file and line number? What module or operate induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can drastically accelerate your debugging course of action.
Some errors are obscure or generic, As well as in Those people circumstances, it’s important to look at the context by which the error transpired. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t neglect compiler or linter warnings both. These generally precede larger problems and provide hints about probable bugs.
Ultimately, error messages aren't your enemies—they’re your guides. Finding out to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood without having to pause execution or action in the code line by line.
A very good logging system starts off with recognizing what to log and at what stage. Prevalent logging degrees include things like DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic info in the course of advancement, INFO for general situations (like prosperous start out-ups), WARN for possible issues that don’t break the applying, Mistake for genuine difficulties, and FATAL in the event the process can’t continue on.
Keep away from flooding your logs with extreme or irrelevant Developers blog data. Far too much logging can obscure significant messages and slow down your method. Focus on critical activities, state variations, input/output values, and critical final decision factors inside your code.
Structure your log messages Obviously and consistently. Include things like context, for example timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Permit you to monitor how variables evolve, what ailments are satisfied, and what branches of logic are executed—all devoid of halting the program. They’re In particular beneficial in generation environments where stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about equilibrium and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify issues, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not merely a technological job—it's a sort of investigation. To correctly identify and resolve bugs, builders ought to solution the process like a detective solving a mystery. This frame of mind can help stop working complex problems into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Consider the signs or symptoms of the condition: mistake messages, incorrect output, or performance problems. Much like a detective surveys a criminal offense scene, acquire as much appropriate facts as you could without having leaping to conclusions. Use logs, take a look at cases, and user reviews to piece with each other a clear image of what’s occurring.
Up coming, type hypotheses. Request oneself: What could possibly be leading to this conduct? Have any adjustments not too long ago been created for the codebase? Has this problem occurred prior to under comparable situations? The aim is usually to slim down opportunities and discover likely culprits.
Then, take a look at your theories systematically. Endeavor to recreate the challenge within a controlled ecosystem. When you suspect a particular function or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code inquiries and let the effects direct you closer to the reality.
Shell out close attention to small particulars. Bugs often cover while in the least predicted locations—similar to a missing semicolon, an off-by-just one error, or a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well hide the true problem, only for it to resurface afterwards.
Finally, retain notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can conserve time for foreseeable future issues and aid Many others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed difficulties in complex techniques.
Publish Checks
Writing exams is one of the best solutions to help your debugging abilities and All round growth performance. Checks don't just help catch bugs early but additionally serve as a safety net that gives you self-confidence when generating improvements towards your codebase. A perfectly-analyzed software is much easier to debug mainly because it allows you to pinpoint exactly where and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily expose no matter whether a certain piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glance, drastically minimizing time invested debugging. Device assessments are Specially beneficial for catching regression bugs—problems that reappear following Beforehand staying mounted.
Subsequent, combine integration assessments and conclude-to-finish tests into your workflow. These enable be certain that different parts of your software perform together effortlessly. They’re specifically helpful for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can tell you which Section of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a feature appropriately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of knowledge By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing test that reproduces the bug might be a robust first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check pass when the issue is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing recreation into a structured and predictable course of action—helping you catch a lot more bugs, speedier plus more reliably.
Take Breaks
When debugging a tricky concern, it’s uncomplicated to be immersed in the condition—staring at your screen for hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging resources is just stepping away. Taking breaks assists you reset your thoughts, minimize stress, and sometimes see The problem from a new viewpoint.
When you are also close to the code for also extended, cognitive tiredness sets in. You could commence overlooking apparent mistakes or misreading code which you wrote just hours earlier. In this point out, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Numerous developers report getting the basis of a difficulty after they've taken the perfect time to disconnect, allowing their subconscious perform within the background.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping absent means that you can return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
Should you’re trapped, an excellent general guideline is usually to established a timer—debug actively for 45–sixty minutes, then take a five–10 minute crack. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, In particular below restricted deadlines, but it in fact leads to more rapidly and more practical debugging Over time.
To put it briefly, using breaks will not be a sign of weakness—it’s a wise strategy. It provides your Mind House to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Just about every Bug
Each and every bug you come upon is more than just A brief setback—It can be a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural problem, each can train you a thing valuable in the event you take some time to mirror and assess what went Erroneous.
Get started by asking yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make more robust coding behaviors transferring forward.
Documenting bugs can be a superb behavior. Maintain a developer journal or preserve a log where you Take note down bugs you’ve encountered, the way you solved them, and That which you uncovered. With time, you’ll start to see styles—recurring challenges or prevalent problems—which you can proactively stay away from.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short produce-up, or a quick knowledge-sharing session, encouraging Other folks avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
A lot more importantly, viewing bugs as classes shifts your attitude from frustration to curiosity. In place of dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce ideal code, but individuals that constantly study from their errors.
In the long run, Every bug you deal with provides a fresh layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It helps make you a far more successful, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be superior at what you do.